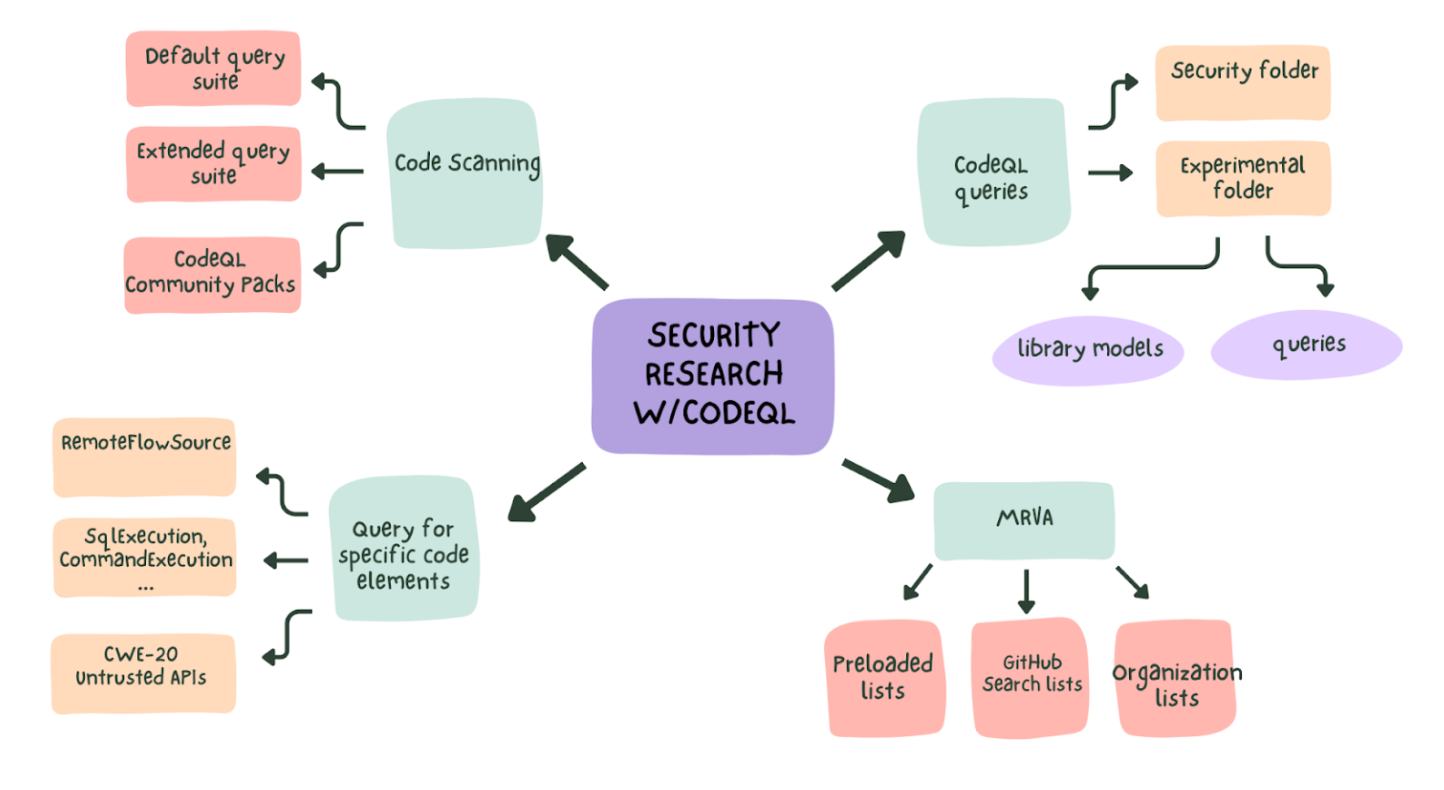
codeql_zero_to_hero part3学习
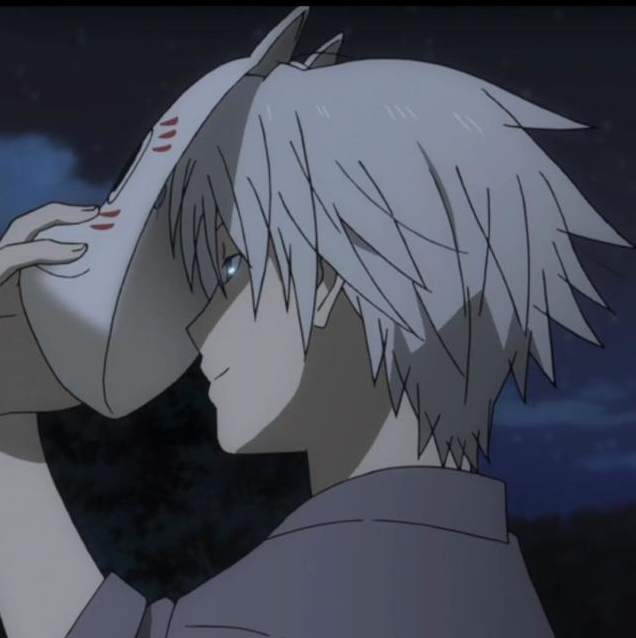
考虑challenge2 中的 sql-injection-flask.py 代码:
1 | from django.db import connection, models |
写如下ql脚本
1 | /** |
首先注解里面的 @kind problem
会返回查询到的结果所在的路径信息。
其次from API::CallNode node
限制了 node 只考虑 API graph 里面的节点,比如说上图中的程序调用了django
这个库,这时候这个库里面的一些方法调用就会被纳入到 API:CallNode
的考虑范围。
在where子句中,使用API::moduleImport("django")
筛选出来自django
库的节点。然后,我们通过.getMember("db").getMember("connection").getMember("cursor")
找到对cursor
的引用。
这将匹配django.db.connection.cursor
。由于在cursor对象上调用execute方法,因此首先需要使用getReturn()
谓词来获取表示创建cursor对象结果的节点,这将返回django.db.connection.cursor()
(注意末尾的括号)。最后,通过getMember("execute")
获取表示execute
方法的节点,并通过getACall()
获取对由execute
节点表示的方法的实际方法调用。
Challenge 1
Find all method calls that are called ‘execute’ and come from the django.db
library
上面 ql 脚本就是 c1 的答案。
Challenge 2
Write a query to find all os.system
method call
找到所有的 os.system
调用,照猫画虎即可
1 | /** |
Challenge 3
Write a query to find all Flask requests
1 | /** |
Challenge 4
Run the query with getAQlClass
predicate
1 |
|
Taint analysis in Codeql
local data flow
顾名思义,只跟踪局部数据流,比如说只考虑一个函数内的 local data flow。
1 |
|
这里的指令用来找到所有不以固定字符串为参数的execute
节点(sink点)
最终结果显示也是如愿找到满足条件的节点。
Global data flow
这种方法会跟踪整个代码库的数据流,这也是大部分情况中所采用的方法,
Challenge 5
Run the local data flow query to find execute
calls that do not take a string literal
就是上面 local data flow 的内容
New taint tracking API
下面为污点分析最新的API配置代码
1 | /** |
需要注意的是通过设定@kind path-problem
,就可以实现看见source
和sink
点之间的路径,接下来我们尝试使用新的污点分析API来实现Challenge 3中的数据流跟踪。
1 | /** |
API::moduleImport("flask").getMember("request").getMember("args").asSource()
简单解释一下这段代码,首先还是通过 API graph 提取目标节点,然后通过 asSource()
将该节点设置为我们要分析的 data flow graph 中的一个source点。
exists(ExecuteCall call | sink = call.getArg(0))}
sink 点的定义就更简单了,直接为cursor.execute
就可以了。
Challenge 6
Run the taint tracking query to find flows from a Flask request to a django.db’s execute
sink
上面即为 Challenge 6 的结果
Variant analysis
简单来说通过一种基本漏洞,尝试去发现类似的其他漏洞,比如说你现在自己审计出来一个漏洞,然后可以尝试通过codeql去建立这个漏洞的规则,然后说不定就可以发现其他相似类型的漏洞在代码库当中。
Source and sink models in CodeQL
sources
codeql 需要有能力去区分一个节点是不是 source,因此这需要提前进行建模,比如说我们前面的flask
模块,这些都已在codeql中集成好了,
1 | API::Node request() { result = |
这里 有预定义好的一些 source,这些source的类型被设置为RemoteFlowSource
,比如说下面的flask.request
1 | private class FlaskRequestSource extends RemoteFlowSource::Range { |
sinks
Codeql 也为每种漏洞类型建立了对应的sink点,比如说 cursor.execute
1 | private class ExecuteMethodCall extends SqlExecution::Range, API::CallNode { |
Security research methodology with CodeQL—approaching a new target
第一步:Quick look with code scanning
先直接开扫就完事了,GitHubSecurityLab/CodeQL-Community-Packs 这里有codeql团队使用的一些queries可供参考
第二步:Run specific queries or parts of queries
<language>/ql/src/Security/
这个文件夹里面有各种漏洞类型的queries,可以针对某种特定漏洞类型先测测
由于这里限制了最大运行数为20,所以需要修改 codeQL.runningQueries.maxQueries 设置,从而提升这个限制:
1. 打开 CodeQL 的设置文件:
• 在 VS Code 中按下 Ctrl + ,(打开设置)。
• 搜索 CodeQL Running Queries MaxQueries。
2. 提高并行查询数限制,比如将其改为 50:
Challenge 7
完成上面的操作就好了
第三步:Find all sources with the RemoteFlowSource type
尽可能的找到所有的 sources
,方法如下:
1 | /** |
成功找到了一些source点,如果想要限制我们扫描source点的范围,可以像Challenge 1中那样,通过getLocation
来限制查询的范围。
Challenge 8
上述内容即为Challenge 8
第四步:Find all sinks for a specific vulnerability type
可以考虑使用Quick evaluation
功能,比如说想查询sql注入的sink点,先进入到python/ql/src/Security/CWE-089/SqlInjection.ql
文件,进入到SqlInjectionQuery
定义的地方
点击下图中isSink
上方的Quick Evaluation
即可开始自动扫描可能的sink点
这里也是成功扫描出来了
还有一种类似获取source点的方法,比如说查询所有SqlExecution
类型的sink点
1 | /** |
第五步:Find calls to all external APIs (untrusted functionality)
CWE-20 Untrusted APIs 可以用来检测外部API是否使用不受信任的数据来源,具体位置如下,运行即可
vscode-codeql-starter/ql/python/ql/src/Security/CWE-020-ExternalAPIs/UntrustedDataToExternalAPI.ql
Challenge 10
Run CWE-20 Untrusted APIs query
如上
Challenge 11
Run MRVA using one of the security queries
MRVA 支持 query 同时运行在多个项目上,可以大幅提高分析效率
https://github.com/GitHubSecurityLab/codeql-zero-to-hero/blob/main/3/11/instructions.md
这里 可以设置扫描的项目
暂时没有大规模需求,先放着吧
Community research
以下是一些C/C++相关的参考资料
- Bug Hunting with CodeQL in Rsyslog
Beginner-friendly step by step process of finding vulnerabilities using CodeQL in Rsyslog by @agustingianni .- Hunting bugs in Accel-PPP with CodeQL by Chloe Ong and Removing False Positives in CodeQL by Kar Wei Loh.
The two researchers worked together to find memory corruption bugs in Accel-PPP. The first article is an in-depth writeup about looking for the bugs and the thought process, writing CodeQL and the challenges they’ve encountered. The second article shows how they refined the CodeQL query to provide more precise results.- Finding Gadgets for CPU Side-Channels with Static Analysis Tools
Research by @pwningsystems and @fkaasan into finding useful gadgets in CPU side-channel exploitation.
Reference
- Title: codeql_zero_to_hero part3学习
- Author: henry
- Created at : 2024-12-17 21:12:04
- Updated at : 2024-12-17 21:14:40
- Link: https://henrymartin262.github.io/2024/12/17/codeql_note_p3/
- License: This work is licensed under CC BY-NC-SA 4.0.